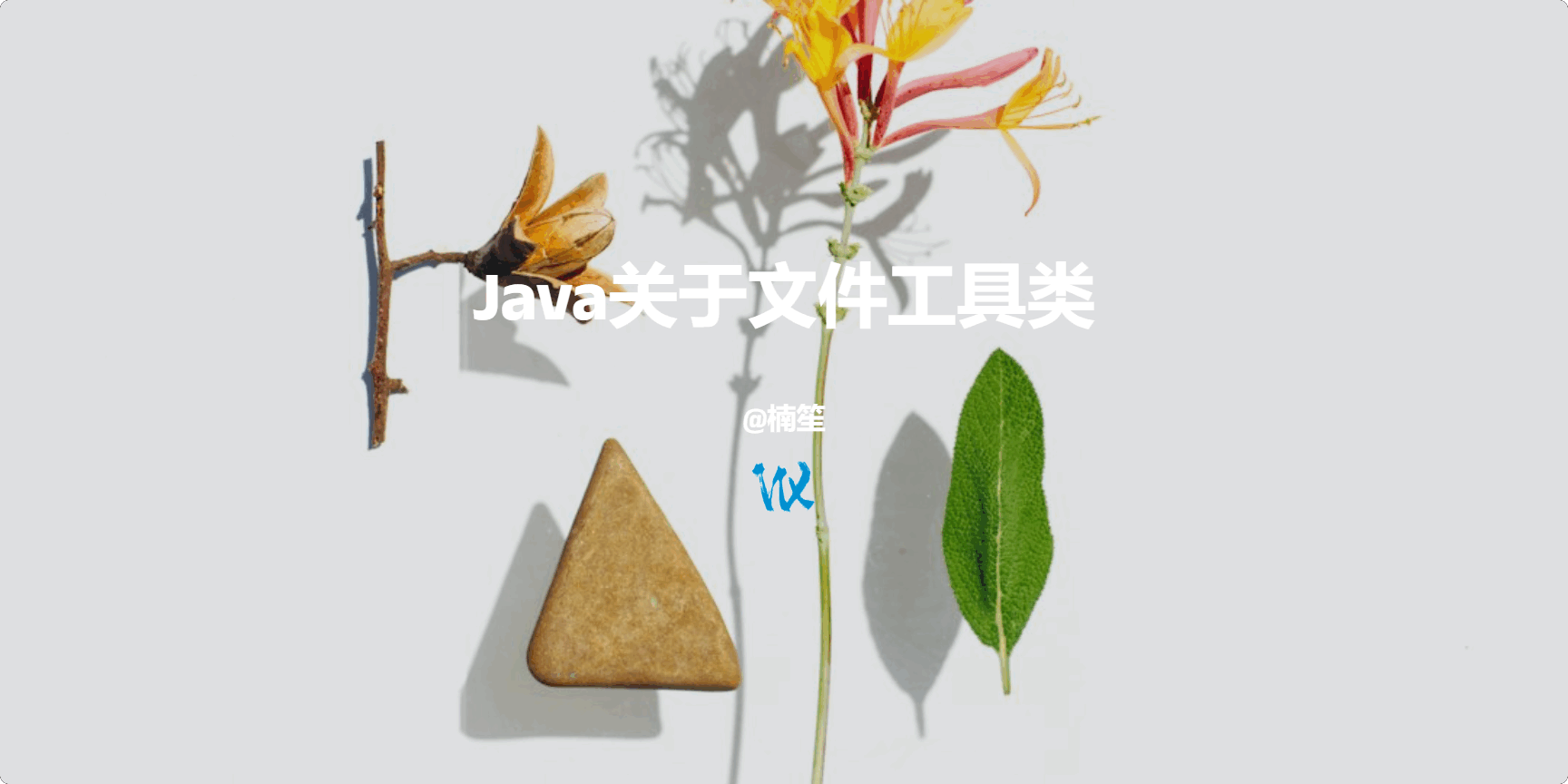
Java关于文件工具类(持续更新)
zip解压
public static void unzip(Path zipFilePath, Path unzipDirPath) throws IOException {
try (ZipInputStream zis = new ZipInputStream(Files.newInputStream(zipFilePath), Charset.forName("GBK"))) {
ZipEntry zipEntry = zis.getNextEntry();
while (zipEntry != null) {
Path newFilePath = unzipDirPath.resolve(zipEntry.getName());
if (zipEntry.isDirectory()) {
Files.createDirectories(newFilePath);
} else {
Files.createDirectories(newFilePath.getParent());
Files.copy(zis, newFilePath, StandardCopyOption.REPLACE_EXISTING);
}
zipEntry = zis.getNextEntry();
}
zis.closeEntry();
}
}
-
zipFilePath是要解压的文件路径
-
unzipDirPath是解压后文件要存放的位置
遍历某个文件夹
/**
* 遍历文件夹下的所有文件
* @param directory
* @return
* @throws IOException
*/
public static List<String> listFilesInDirectory(String directory) throws IOException {
List<String> filePaths = new ArrayList<>();
Files.walkFileTree(Paths.get(directory), new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attrs) throws IOException {
filePaths.add(file.toString());
return FileVisitResult.CONTINUE;
}
@Override
public FileVisitResult visitFileFailed(Path file, IOException exc) throws IOException {
return FileVisitResult.CONTINUE;
}
});
return filePaths;
}
-
directory是文件夹路径
路径,文件名,文件扩展名相关操作
/**
* 获取路径中的文件名
* @param filePath 文件名
* @return
*/
public static String extractBetweenLastSlashAndDot(String filePath) {
int lastSlashIndex = filePath.lastIndexOf('/');
int lastDotIndex = filePath.lastIndexOf('.');
if (lastSlashIndex == -1 || lastDotIndex == -1 || lastDotIndex <= lastSlashIndex) {
return ""; // 无效情况处理
}
return filePath.substring(lastSlashIndex + 1, lastDotIndex);
}
/**
* 获取最后一个点之前的所有字符串
* @param str
* @return
*/
public static String getStringBeforeLastDot(String str) {
int lastDotIndex = str.lastIndexOf('.');
if (lastDotIndex == -1) {
return str; // 如果没有找到点,返回原始字符串
}
return str.substring(0, lastDotIndex);
}
/**
* 获取最后一个/之后的所有字符串
* @param str
* @return
*/
public static String getStringAfterLastSlash(String str) {
int lastSlashIndex = str.lastIndexOf('/');
if (lastSlashIndex == -1) {
return str; // 如果没有找到斜杠,返回原始字符串
}
return str.substring(lastSlashIndex + 1);
}
/**
* 获取文件扩展名
*/
public static String getFileType(String filePath) {
if (filePath == null || filePath.isEmpty()) {
return ""; // 如果文件路径为空,返回空字符串
}
int lastDotIndex = filePath.lastIndexOf('.');
if (lastDotIndex == -1 || lastDotIndex == filePath.length() - 1) {
return ""; // 如果没有找到点或点在最后,返回空字符串
}
return filePath.substring(lastDotIndex + 1);
}
- 感谢你赐予我前进的力量
赞赏者名单
因为你们的支持让我意识到写文章的价值🙏
本文是原创文章,采用 CC BY-NC-ND 4.0 协议,完整转载请注明来自 楠笙
评论
隐私政策
你无需删除空行,直接评论以获取最佳展示效果